The subset sum problem is a common question in computer science. This problem is about finding a subset of numbers that add up to a target sum. Let’s explore this problem and solve it using Java.

Credit: www.interviewbit.com
Understanding the Subset Sum Problem
Imagine you have a list of numbers. You need to find a group of these numbers that add up to a specific value. For example, if the list is [1, 2, 3, 4, 5] and the target sum is 9, the subset [4, 5] adds up to 9.
Why Is It Important?
This problem is important in many areas. It is used in resource allocation, cryptography, and other fields. Learning how to solve it can help in understanding more complex problems.
Approach to Solve the Problem
There are several ways to solve the subset sum problem. We’ll look at two methods: recursive and dynamic programming.
Recursive Method
The recursive method is simple to understand. It checks if a subset can be formed with or without the last number. Here’s the code for the recursive method:
public class SubsetSum {
public static boolean isSubsetSum(int[] set, int n, int sum) {
if (sum == 0)
return true;
if (n == 0 && sum != 0)
return false;
if (set[n - 1] > sum)
return isSubsetSum(set, n - 1, sum);
return isSubsetSum(set, n - 1, sum) ||
isSubsetSum(set, n - 1, sum - set[n - 1]);
}
public static void main(String[] args) {
int[] set = { 3, 34, 4, 12, 5, 2 };
int sum = 9;
int n = set.length;
if (isSubsetSum(set, n, sum))
System.out.println("Found a subset with given sum");
else
System.out.println("No subset with given sum");
}
}
In this code, `isSubsetSum` checks if the subset exists. It reduces the problem by checking subsets without the last number.
Dynamic Programming Method
The dynamic programming method is more efficient. It uses a table to store results of subproblems. Here’s the code for the dynamic programming method:
public class SubsetSumDP {
public static boolean isSubsetSum(int[] set, int n, int sum) {
boolean[][] subset = new boolean[sum + 1][n + 1];
for (int i = 0; i <= n; i++)
subset[0][i] = true;
for (int i = 1; i <= sum; i++)
subset[i][0] = false;
for (int i = 1; i <= sum; i++) {
for (int j = 1; j <= n; j++) {
subset[i][j] = subset[i][j - 1];
if (i >= set[j - 1])
subset[i][j] = subset[i][j] || subset[i - set[j - 1]][j - 1];
}
}
return subset[sum][n];
}
public static void main(String[] args) {
int[] set = { 3, 34, 4, 12, 5, 2 };
int sum = 9;
int n = set.length;
if (isSubsetSum(set, n, sum))
System.out.println("Found a subset with given sum");
else
System.out.println("No subset with given sum");
}
}
In this code, `isSubsetSum` uses a table to keep track of possible sums. It updates the table to find the subset.
Comparing the Methods
Both methods have their pros and cons. The recursive method is simple but can be slow for large sets. The dynamic programming method is faster but uses more memory.
Method | Advantages | Disadvantages |
---|---|---|
Recursive | Easy to understand | Slow for large sets |
Dynamic Programming | Efficient for large sets | Uses more memory |
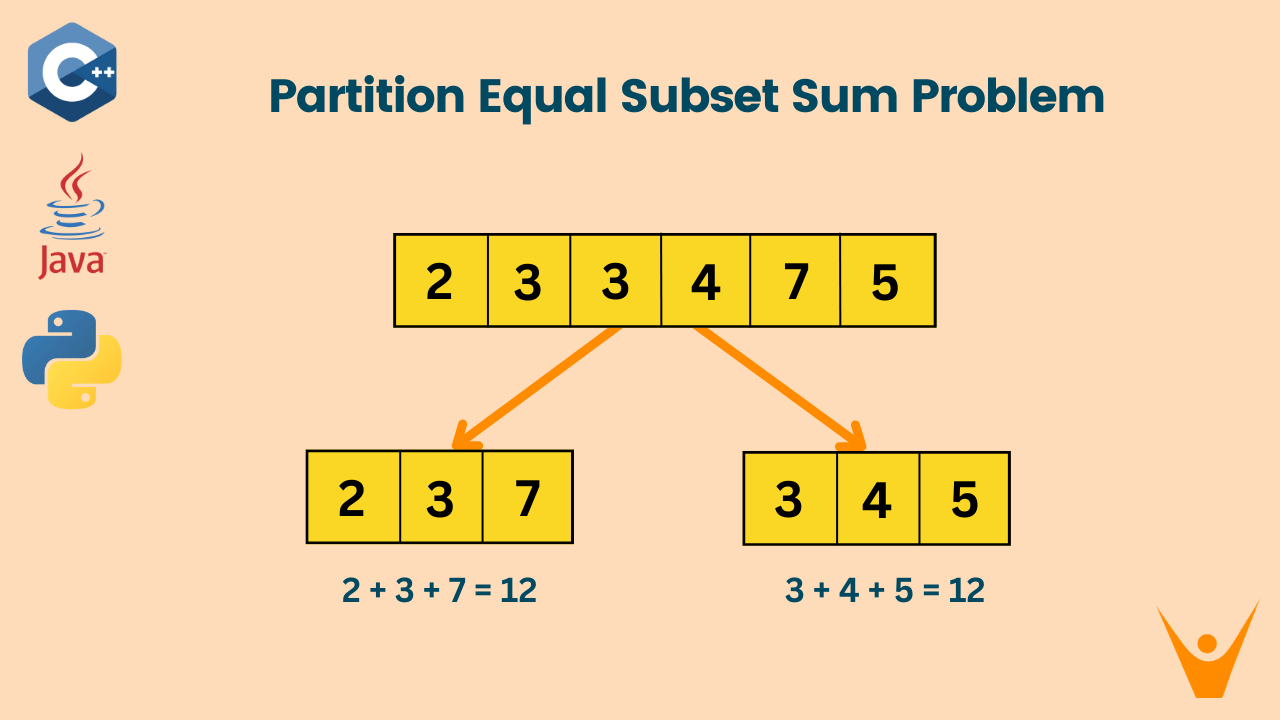
Credit: favtutor.com
Choosing the Right Method
Choosing the right method depends on your needs. If you have a small set, the recursive method is fine. For large sets, the dynamic programming method is better.
Frequently Asked Questions
What Is The Subset Sum Problem?
The Subset Sum Problem is about finding a subset whose sum equals a given number.
Why Is The Subset Sum Problem Important In Java?
It helps solve real-world problems, like resource allocation and finance management in Java applications.
How Do You Solve The Subset Sum Problem In Java?
Use recursion, dynamic programming, or backtracking to solve the Subset Sum Problem in Java.
Can The Subset Sum Problem Be Solved In Polynomial Time?
No, it is an NP-complete problem, so no polynomial-time solution is known.
Conclusion
The subset sum problem is a useful problem to understand. Solving it in Java helps learn important concepts. Both recursive and dynamic programming methods are helpful. Choose the method that fits your problem size.
Further Reading
If you want to learn more, there are many resources available. Books on algorithms and data structures often cover the subset sum problem. Online tutorials and courses can also be helpful.